Checkouts
Chekouts are the simplest way to collect payments. They are used for one-time payments, such as a purchase of a product or service or donations. In this guide you'll learn how to create a basic checkout for the most common use case. You can then extend it to more complex scenarios.
Setup
Create a project
Login to your dashboard, go to the Projects section and click on Create Project:
Make sure to select the appropiate environment for your project. If you select Production you should use the production credentials for the payment provider. Using sandbox credentials in production will cause errors. Same as the other way around, sandbox provider credentials should only be used in a project with the environment set to Sandbox.
Add credentials
Go to the project settings and choose the providers you want to use. For now, we don't need the webhook_secret, we'll show you how to use it later. For this demo, we'll use Stripe.
Where to get the credentials:
We assume you already have an account created within Stripe and that you understand how to setup and differentiate between sandbox and production configurations. If not, you can read more here.
- Go to your Stripe dashboard and select the account you want to use.
- Go to Developers > API Keys and click on API Keys.
- Copy the Publishable Key and Secret Key and paste them in the corresponding fields in the Vexor dashboard in the Project details as shown before.
As you can see in the video, we have selected Sandbox as the environment (a sandbox account). So our Vexor project will use the sandbox credentials for Stripe. If you select Production you should use the production credentials for Stripe.
Let's code
Create the app
In this example we will implement Vexor Checkout in a Next.js bare project but the same logic can be applied to any other framework.
Run:
to create a new Next.js project.
Install the SDK and instantiate it
Run:
to install the SDK.
Create a file, typically named vexor.ts
instantiate it as follows:
As a convention, it is recommended to place this file inside a lib
folder, located inside the src
folder as shown below.
Let's build a simple component for the products
Create a file named product-card.tsx
inside a src/components
folder and add the following code:
Import the component in the page and define some products
Ideally this component will be used in a page that lists all the products. For demonstration purposes, we will just define some products in the main page.tsx
file.
Remove the default boilerplate code from the page.tsx
file and replace it with the following code:
Run your app locally, you should see something like this:
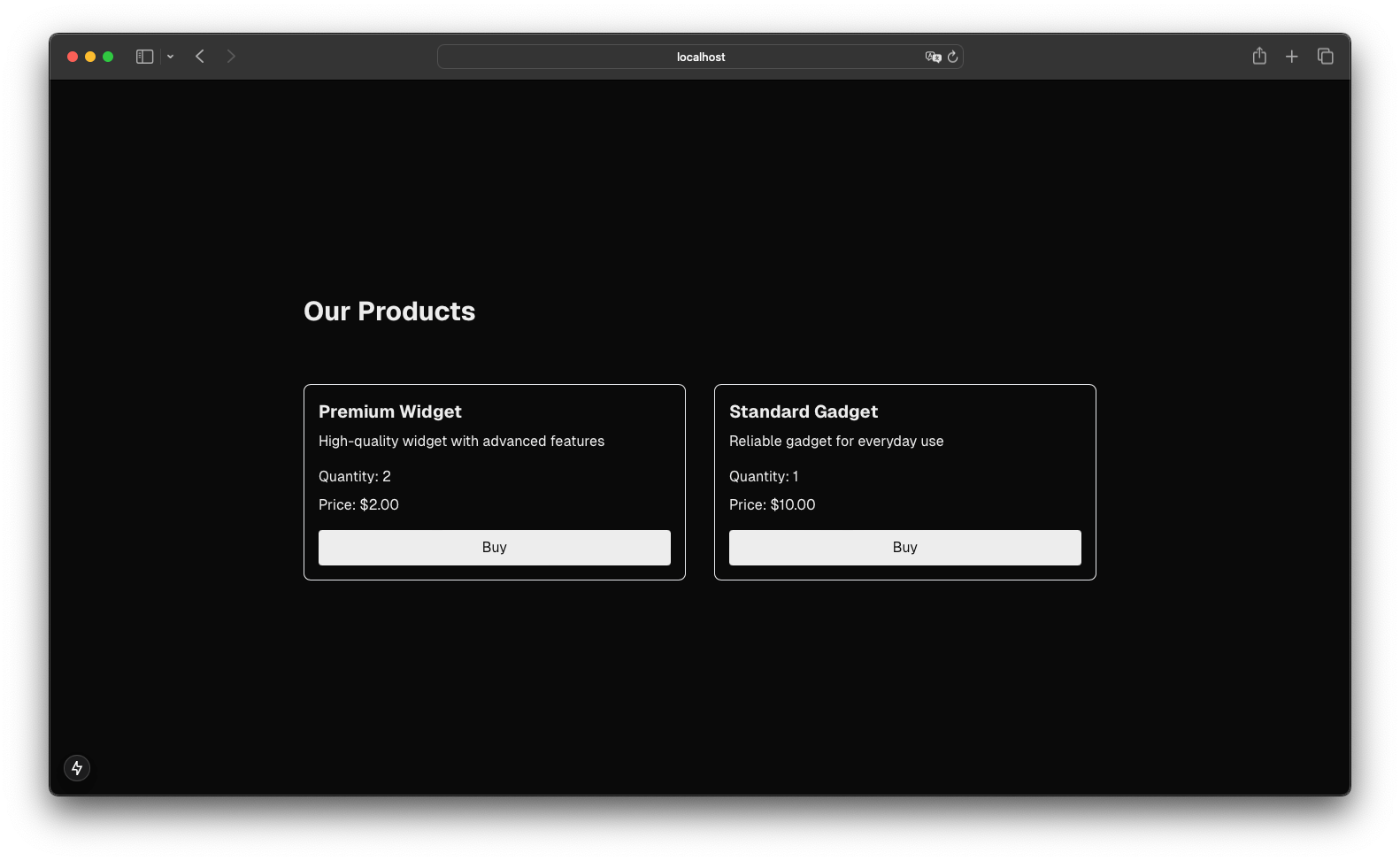
Great! ✨ Now let's complete the purchase logic...
Use the Vexor SDK to create the purchase
In our product-card.tsx
file, we have a handlePurchase
function that is currently empty:
Import vexor, VexorPaymentResponse and useTransition hook
The useTransition hook from react is a good approach to handle the logic and loading state of the purchase. We will also import the VexorPaymentResponse type to handle the response of the purchase (recommended).
Complete the handlePurchase function
Let's complete the handlePurchase
function to create the purchase and redirect the user to the payment page. As you can see, we need to use isPending and startTransition from the useTransition hook to handle the loading state and the purchase logic.
And also let's make the button disabled when the purchase is pending and show a loading message:
The vexor.pay.stripe
function is used to create the purchase. You can use any other provider by calling the appropriate function, for example vexor.pay.mercadopago
, vexor.pay.talo
or vexor.pay.paypal
. Or the generic vexor.pay
method. You can read more about the pay methods here.
We use stripe as the payment provider in this guide, but you can use any other provider. Just make sure to complete the provider credentials in your Vexor project.
That's it! Your product-card.tsx
file should now look like this:
Test the purchase
Run your app locally and test the purchase by clicking on the "Buy" button. You should be redirected to the payment page.
Congratulations! 🎉
You have now a basic checkout working. You can extend this logic to more complex scenarios, for example, by adding a cart and a checkout page.
Is this all we need? Yes and no. We have a working checkout, the user can now pay for the products and we get the money after the purchase, but we don't have a way to know if the purchase was successful or not. There are two more things that we recommend you to do:
-
Create the success and failure pages. By default, vexor will redirect to
yourapp.com/success
oryourapp.com/failure
based on the purchase result. You can build a UI for this pages or use the default ones. If you want to customize the redirect urls, you can read more about redirect urls here. -
Add a webhook (Yes, this is important 🫡) to your project to know when the purchase is successful or not. You can read more about webhooks here.
Here you have a video showing the complete process we have done in the last steps of this guide to build the UI of the checkout:
👀 Example videos of the complete process may be outdated and you may find slight differences in the syntax, don't worry, the logic is the same... but now it's better 🚀 We'll update the videos soon.