Webhooks
Webhooks allow you to be notified about operations events. This guide will walk you through creating a basic webhook system using Vexor.
Setup
Webhooks are requests sent to a specific URL when an event occurs. For example, when a subscription is created, updated, renewed or cancelled or when a payment is successful or not. These requests are triggered by the payment provider and sent to the URL you specify to them.
Define the webhook URL
In production environments, you can set the webhook directly as your domain. For example, if you have a domain https://my-app.com
, you can set the webhook URL to https://my-app.com/api/webhooks
or any other route you want.
In development environments, webhooks cannot be set to make a request to localhost, so you need to set the webhook URL to a public URL. In this case, you can use ngrok, cloudflared or any other tunneling service to create a public URL for your local development environment.
In this example, we will create a public URL for our local development environment using cloudflared. Again, if you are working on a production environment, you can skip this step since you will use your production domain instead.
You need to install ngrok or cloudflared to use tunnels. You can find more information about how to install cloudflared for this example here.
Assuming you're working on a local development environment in http://localhost:3000
, run the following command in your terminal to create a public URL:
This will create a public URL and print it in the terminal. Copy that URL, it will be used in the next step.
Set the webhook URL in the provider
You'll need to set the webhook URL in the application settings of the provider you are using.
- Go to your Stripe dashboard and select the account you want to use.
- Go to Developers > Webhooks and click on Add Endpoint.
- Paste the webhook URL you created in the previous step, append the route you want for the webhook in the URL (in our example we chose
/api/webhooks
) and click on Add an Endpoint. You can also choose the option "Test in a local environment" provided by Stripe to test the webhook. However, if you are integrating other providers, a better approach would be to use the tunneling service to create a public URL for all of them. - Paste the endpoint URL in the URL field and write any description you want for the webhook in the Description field.
- Select the events you want to be notified about and click on Save. Normally you'll want to be notified about the following events:
- checkout.session.completed ππ» This event is triggered when a checkout session is completed. In other words, when a customer completes the checkout process after paying for a product or service.
- invoice.payment_succeeded ππ» This event is triggered when an invoice payment is successful. Normally this is triggered after the subscription is created and paid and also when the subscription is renewed.
With these two events, and a good logic in your app, you can build a basic yet solid payment system.
As you can see in the video, we have pasted the URL that we created in the previous step. But we have also set a specific route for the webhook in the URL /api/webhooks
. This is the endpoint you need to create in your app, this is where the webhook will be sent and where you'll add the logic to handle the webhook events.
π Don't worry, we will see how to create this endpoint later.
Complete the webhook secret in the Vexor project
In the previous step, you created the webhook URL in the provider and selected the events you want to be notified about. Now you need to complete the webhook secret in the Vexor project.
The webhook secret is a random string that is used to verify the authenticity of the webhook request. Every provider provides a webhook secret after you create the webhook URL as we saw in the previous step.
Where do I find the webhook secret?
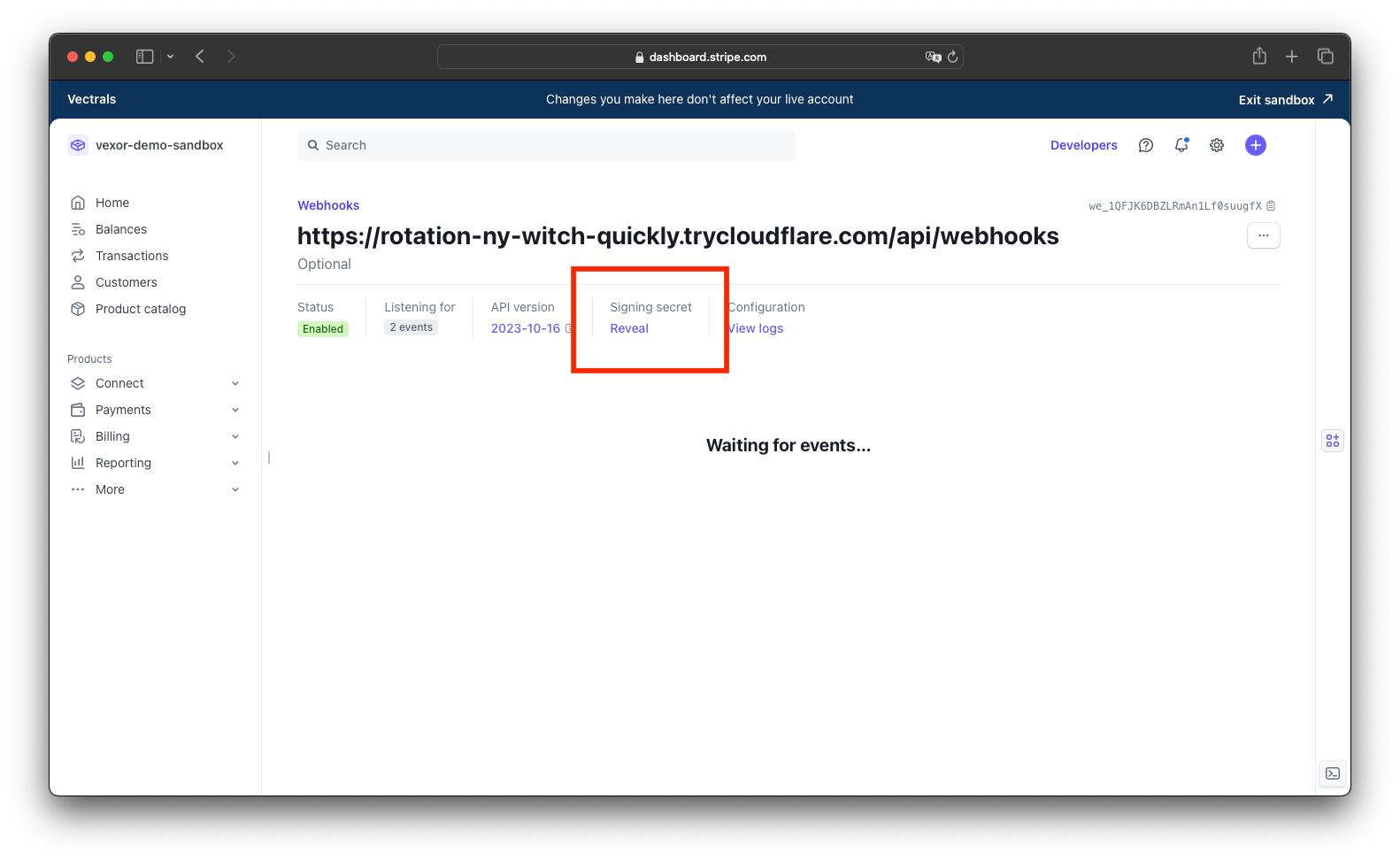
In the following video example, we're setting up the webhook secret provided by Stripe:
Let's code π
Create the app
In this example we will implement Vexor webhooks in one of the previous examples. We are going to clone the app we created in the Subscriptions Guide but the same logic can be applied to any other application.
Clone the basic
branch of the subscriptions repo:
This branch contains the basic implementation of the subscriptions app without the webhooks implementation yet so we can add it in the next steps. Once you have cloned the repo, open it on your code editor and install the dependencies:
Create the api folder and the webhooks route
-
Create a folder named
/api
inside the/app
folder. Remember that we are following the Next.js convention for the folder structure, if you are using another framework, you can use the appropriate folder structure. -
Inside the
/api
folder, create another folder named/webhooks
. -
Inside the
/webhooks
folder, create a file namedroute.ts
. -
Let's complete the
route.ts
file with the following code:
That's it! You have now a basic webhook endpoint. Easy, right? π€
Test the subscription
- Run your app locally and test subscribing to a plan by clicking the "Subscribe" button. You should be redirected to the payment page for the subscription.
- Complete the payment and you should see the webhook response in the console where you ran your app.
Congratulations! π
You have implemented a basic webhook system. You can extend this logic to more complex scenarios. The important thing to remember here is that we are already receiving the payment from the user and the webhook events from the provider, now you can add any logic you want in the POST
function to handle custom logic for your use case.
Here you have a video showing the complete process we have done in the last steps of this guide to build the webhooks logic:
Use the identifier to track the payment
When you create a payment in your application, Vexor generates a unique identifier
for that operation. This identifier is crucial for tracking - you can store it in your database when the payment is initiated, Vexor will include this same identifier in the webhook response. This makes it super simple for you to match incoming webhook events (like successful payments or declines) with their corresponding payment operations in your application.
π Example videos of the complete process may be outdated and you may find slight differences in the syntax, don't worry, the logic is the same... but now it's better π We'll updaΓ₯te the videos soon.
Yeah, I know, I made a typo in the video, it's "any" not "ny" π